strings
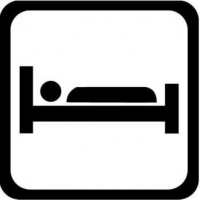
I have a variable that holds a telephone number, including the hyphens (-). The problem is that the device that I'm goin to sen the string, needs the numbers one next to each other, without the hyphens.
If there is someone that knows how to go arround this, I'll be glad to listen.
I tried this, num_mem3=remove_string (TempDial, '-', 1), but is not working.
Please advice...
Thanks
If there is someone that knows how to go arround this, I'll be glad to listen.
I tried this, num_mem3=remove_string (TempDial, '-', 1), but is not working.
Please advice...
Thanks
0
Comments
The way Remove_String works is that it removes UP TO AND INCLUDING what you're looking for starting from a specific character index (the third parameter.) So, by replacing the third parameter with a FIND_STRING, which will return where what you're looking for is, it'll remove the string/char that we want to remove (the second parameter).
Now, here's another way of doing it with a function that's been floating around for quite some time. Just as simple to use also.
As you see, it's your choice on how you wish to skin the cat.
With this, num_mem3 will equal everything up to and including the dash. There are lots of ways to get rid of the dash, heres one:
So lets say this is your string '612-445-1234'
The ATOI function will convert only ASCII representations of numbers to an integer value, any non-number character (in this case the dash) will be dropped.
This function will return '6124451234'
If you are going to be sending it strings with more then two dashes this function will not work, but it should give you a general idea.
this will eliminate any characters that are not a number
My original include was from one of the forum members here - the name on it in Inter (VCD). I have adapted and modified the heck out of it since, but you can still find the original here someplace.
Thanks a lot for all the info... appreciated
Quick side note: since NS2 does not show functions properly when using #INCLUDE (a big gripe of mine), you can put any of your functions in the Netlinx.axi (C:\Program Files\Common Files\AMXShare\AXIs\Netlinx.axi) and it'll pop up everytime you start to type it.
Thanks for posting this it helped me out with what I am currently working on