If statements in FOR loops
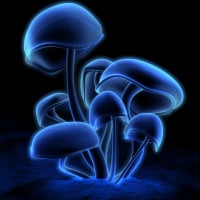
G'day all,
Does anybody know which has greater overhead?
For(x = nNumber; x; x--)
{
//Do stuff
If(x < nSmallerNumber)
{
// Do less stuff
}
}
Or this
For(x = nNumber; x; x--)
{
//Do stuff
}
For(x = nSmallerNumber; x; x--)
{
//Do stuff
}
In the first example you only have the overhead of one for loop but a conditional statement that has to be processed nNumber times.
The second example has the overhead of two for loops but no conditional.
I seem to recall being told that FOR loops in NetLinx are a bit strange.
Cheers
Mush
Does anybody know which has greater overhead?
For(x = nNumber; x; x--)
{
//Do stuff
If(x < nSmallerNumber)
{
// Do less stuff
}
}
Or this
For(x = nNumber; x; x--)
{
//Do stuff
}
For(x = nSmallerNumber; x; x--)
{
//Do stuff
}
In the first example you only have the overhead of one for loop but a conditional statement that has to be processed nNumber times.
The second example has the overhead of two for loops but no conditional.
I seem to recall being told that FOR loops in NetLinx are a bit strange.
Cheers
Mush
0
Comments
I can't imagine it would make much difference. Just stay away from nested for loops if you can. There isn't much point in optimizing a for loop, since whatever you do could only have a miniscule effect on overall execution. You don't show the 'do stuff' code and that might influence my choice, but I would write it the first way since its more standard practice. But it depends on what you are trying to do as there are times when two for loops makes more sense.
Paul
Why no nested loops?
In Netlinx land, your master will pretty much stop all processes while doing the loops. I've never had anything get lost or dumped during a particularly spendy nested loop (as far as I know) but I suppose the possibility is there.
Like most things, it's a tool and you have to know when to use it and what it costs to do so.
Jeff
As you can see, I added a couple more approaches. All approaches are fairly close, so it might be dependent on the code running within.
The first alt test uses a little less efficient for loop, but by cutting out the redundant passes through the low range, you pick up more speed than you sacrifice. This is of course dependent on the size of the redundant portion of the loops.
The second test uses two efficient for loops to accomplish the same thing, but if you need to use the x value of the for loop, this might not be more efficient.
Hope this helps,
Jeff
something like
Not sure what you are asking me to test here? I you are asking that I perform the various tests within a loop to 5000, I can do that when I get back to the office, but I am thinking that the results will be something in the area of currentTestResult * 5000. Or do you have a couple of different ways to parse what you are describing?
Jeff
for (i = 1; i<= 10000;i++)
{
for (j = 1; j<= 10000;j++)
{
// do some text searching
}
}
Although an array of 10000 bytes isn't very big, if you are processing this nested for loop (in a text search for example) you are now running it 100,000,000 times. The cpu time needed increases as the square of the input so things can get slow really fast. If you added another for loop it would run 1,000,000,000,000 times. See how long 3 nested for loops takes. I think you will likely be waiting a long time for it to complete.
Paul
Thanks for that Jeff!
Very interesting result, your second alternate represents a time saving of up to ≈31%
Whilst this is not significant when talking about test passes that take only ≈20ms it could represent significant savings in very large programs.
How did you resolve your test results? Are you using timelines?
Thanks again
Mush
Thanks for clarifying Paul, I thought there was another NetLinx idiosyncrasy that I didn't know about.
I regularly use nested loops but never anywhere near that order of magnitude.
Cheers
Mush
Yes, I am using timelines as they seemed to have the highest resolution. I also decided to keep the testing to a small enough run time to distinguish results without (hopefully) introducing other processor overhead into the mix.
Here is a link to the code I am using (I think it's current with what I have): http://www.amxforums.com/showthread.php?5297-Benchmark-Code&highlight=benchmark
I will post the latest code I am using, when I have a chance, if it is different.
Jeff
Thanks Jeff!