Resetting a count
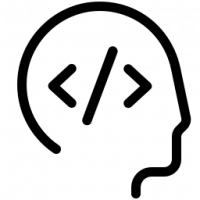
There's gotta be a better way than this...
I've never really thought about it - but I am now.
What's more processing power, a mathematical equation to creating a "ceiling" or an evaluation?
i_theNumber ++; if(i_theNumber == 4) i_theNumber = 1;
I've never really thought about it - but I am now.
What's more processing power, a mathematical equation to creating a "ceiling" or an evaluation?
0
Comments
Are you trying to make a for loop?
or, if you want to go from 1-4:
If this is what you are asking, I can try to run it through a test later today.
Jeff
I just didn't know if there was a better way of doing it without the comparison of saying, "if you've reached the max, reset yourself back to the beginning." It just doesn't seem very streamlined is all.
Usually greater than would be used rather than equality...unlikely there should be a bug to affect the variable or a cosmic ray will hit the right place and cause the variable to be some random value but who knows? things happen
I suppose you could just use the overrun of an integer.
65535/4 parts and just keep adding them up. the overrun will do the reset for you.
counter++
counter band 1 for the first letter
counter band 2 for the second letter
counter band 3 for the third letter
That should work... binary is
00000000
00000001
00000010
00000011
00000100
00000101
00000110
00000111 the repeat seems to happen naturally
Paul
I like that . . . I thought about that briefly, but am unsure at this moment why i disregarded it. With a clearer head, I'll stick with that approach.
I know this doesn't answer your question but here's how I did it..
Work very well for me.